We’ve had a third party building and managing our native apps for several years and they’re doing a terrible job. We wanted to get rid of them, but weren’t ready to part with the app experience, which is important to our most committed mobile users. We came up with two alternative solutions: build our own native app, or build a Progressive Web App (PWA). I argued that there was no reason we couldn’t do both, since the PWA would only be an enhancement to our existing mobile website and also be a low level of effort to implement. I was only half right. I underestimated the level of effort.
Theoretically, building a PWA is a piece of cake. You need the following:
- A secure HTTPS connection
- A web manifest file with links to the app icons, the site name and description, the start url for the PWA, and the PWA’s scope.
- A service worker, which is created with a JavaScript file placed at the root of your PWA.
- JavaScript to register the service worker and control the behavior of the PWA, for example the “Add to Home Screen” (A2HS) functionality and caching of assets for offline use.
- The graphics for the icons referenced in the manifest.
- A meta tag in the document head specifying the color for the app’s address bar (optional).
If you are working on a small site that has a valid SSL certificate, then this is a truly an easy project. If you are in an enterprise environment working on a large e-commerce site where you don’t have access to the site’s root directory, and in which only the production version of the site is served over valid HTTPS, then it’s far from simple.
As I struggled through the process I found very little help with the issues I was having. Typical of the documentation for a fairly new technology, there was no help for when things didn’t go right and few suggestions for workarounds. By the second day of working on this, every search I did to find solutions to error messages and other roadblocks produced Google result pages full of links I’d already visited and no new insights. I’m documenting my experience here in case anyone else can benefit from it, and because I’ll probably forget all the gotchas myself and I don’t want to have to go through it all again in the future. Unlike the rest of the documentation on the subject, I’m going to focus on what goes wrong and what to do about it, since if everything goes well you don’t need to scour blog posts from random developers. You can simply read the few paragraphs Google or MDN provide and be on your way.
I began by trying to make this work in the Dev environment. I reasoned that would be my best strategy, since in Dev I had access to the entire site, including the root directory, the aspx file containing the code for the document head for every page in the site, and control of the IIS server, which was my localhost. I put everything I needed into the site (.NET) and built and ran it. It didn’t work.
Chrome browser has a feature called “Lighthouse” in its dev tools. Lighthouse reports on the status of your PWA, if you have one.
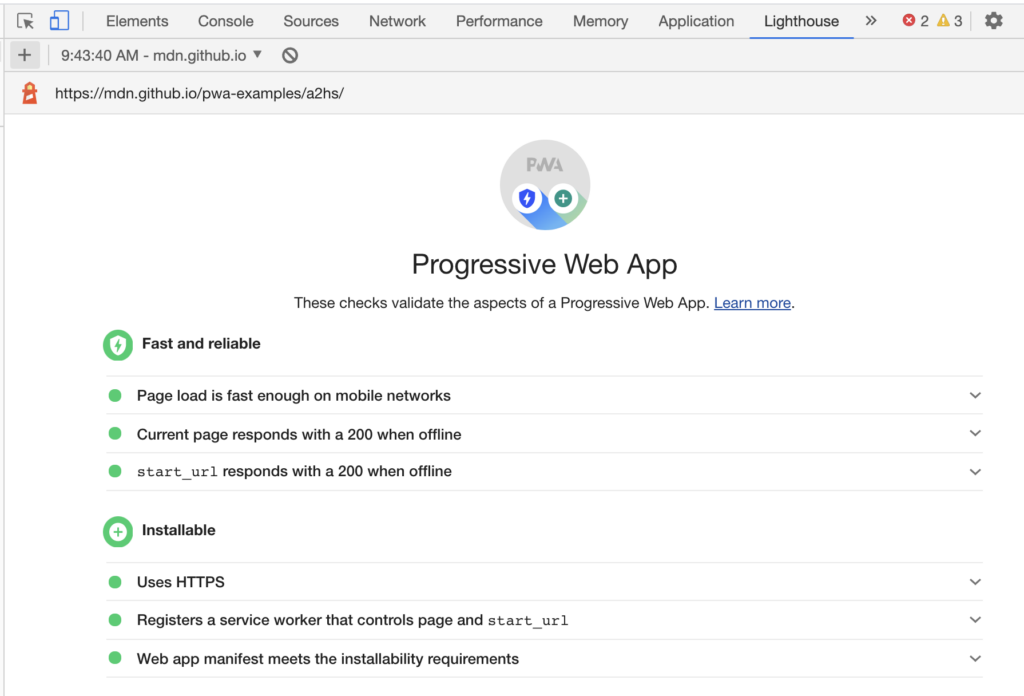
In addition, if you click the “Application” tab in the dev tools panel, you can see “Manifest” and “Service Workers” at the top of the side panel, if they are active.
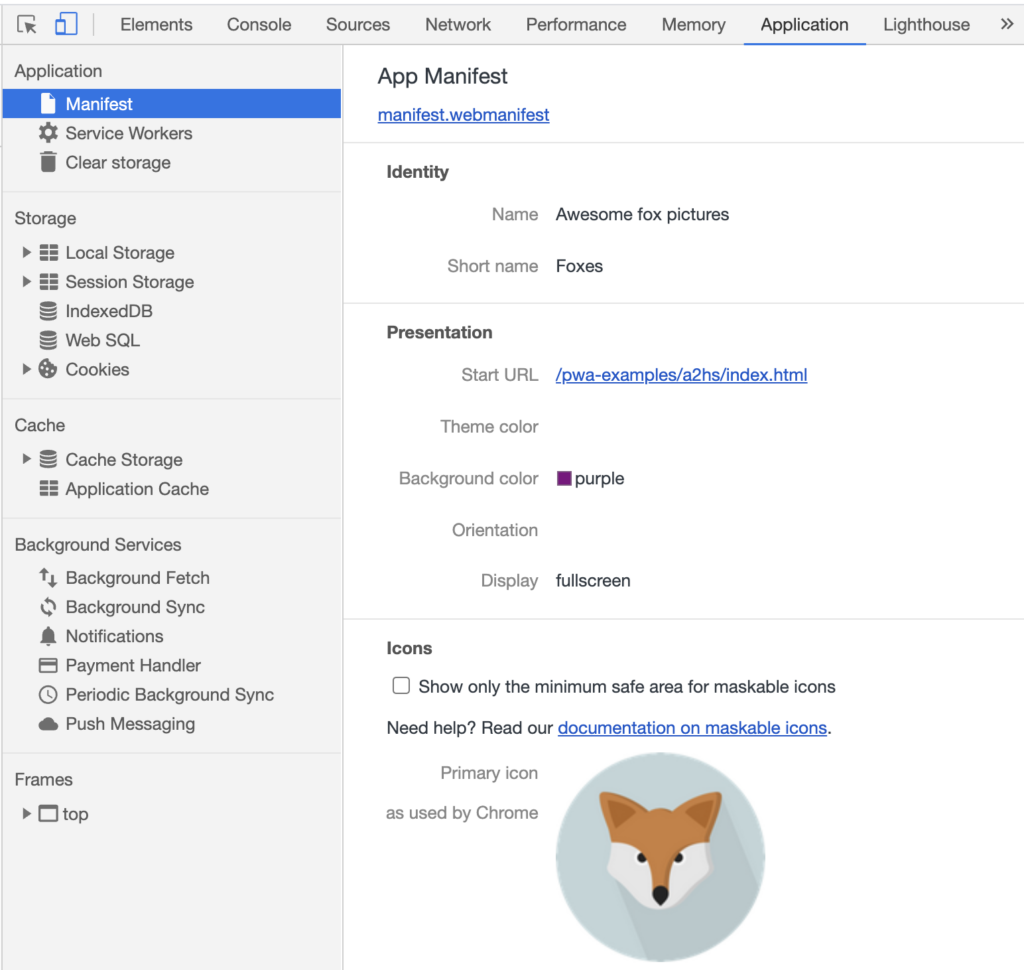
Below the manifest is the “Service Worker” link, and clicking that allows you to interact with your service worker:
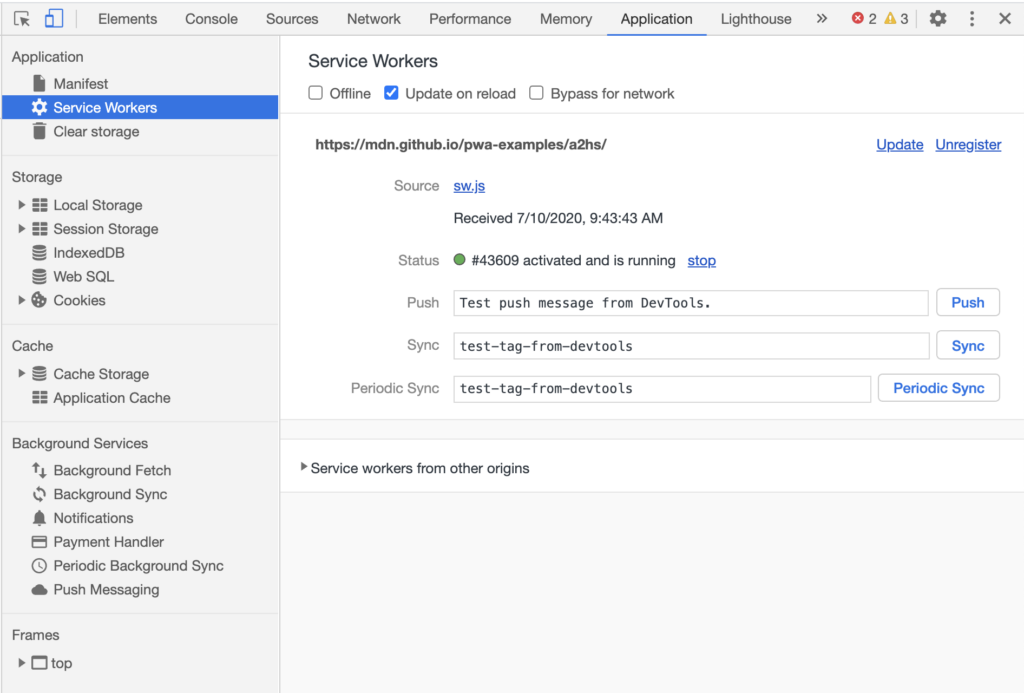
Problem 1: PWAs require HTTPS
My dev server didn’t have HTTPS. There is an SSL certificate in place, but it wasn’t recognized as valid. I tried launching Chrome from the command line so I could invoke the “–ignore-certificate-errors” flag, but that didn’t work. The inspector reported that HTTPS was in place and valid, but deep down it knew it wasn’t and the service worker didn’t work. There was no error in evidence, just a complete failure of the PWA to actually do anything.
TL;DR
If there isn’t a lock icon beside your URL your PWA won’t work.
Problem 2: The service worker script has to be at the root level of your app.
The second problem I had was running the service worker .js file from the root. Service workers only have scope up to the directory they reside in and not above. If you need your service worker to affect your entire site, it’s got to be in the site root, i.e. https://mysite.com/sw.js. This was no problem in the Dev environment where I had root access, but since I had to move to QA or Prod to test with HTTPS, root level was not available to me there without checking code into a release. While that was a possibility, the time scale was too long for the many iterations I would need on the file’s code.
We have a CMS that allows us to check code into the Prod script folders without having them be part of the web project (i.e., they could go to Prod without a build), so that was useful, but I couldn’t get them to root from there. It turns out I didn’t have to.
Our site uses Akamai’s front-end optimization program. As part of that package they inject code into the head of our document that creates a service worker at our root. That code then goes on to collect all other registered service workers on the site and imports them into itself, resulting in my service worker being hoisted to root, and having its scope programmatically reset to root. This was pure luck.
Knowing that this can be done means that sites without this service could do something similar by having a single master service worker at root whose only purpose is to hoist service workers from subdirectories, so that those service worker scripts can be modified outside of the release cycle. Below is the relevant (abbreviated) excerpt:
navigator.serviceWorker.controller) {
var u = navigator.serviceWorker.controller.scriptURL;
u.includes("/akam-sw.js") || u.includes("/akam-sw-preprod.js") || u.includes("/threepm-sw.js") || (aka3pmLog("Detected existing service worker. Removing and re-adding inside akam-sw.js"),
s = new URL(u,window.location.href),
e.then(function() {
navigator.serviceWorker.getRegistration().then(function(e) {
m = {
scope: e.scope
},
e.unregister(),
d()
})
}))
}
TL;DR
Your service worker script has to reside at the root of your app, but it can be hoisted there by another service worker with root scope.
Problem 3: The manifest has to be served from the same domain as your site.
Service worker specs say that the manifest file should have the .webmanifest extension, but that .json can be used instead. Our CMS file copy program wouldn’t upload documents with either of these extensions. The first thing I tried was hosting it at a CMS and linking it in the body, which is how I found out that there is a same-domain policy for the manifest. In other words, the manifest has to live at your own site or it violates CORS policy.
I tried adding it as a data URI, with and without URL encoding. Technically that worked, but my PWA still didn’t. Finally, I realized that I could simply change the file extension to .js and upload it via the CMS without any problem and the browser would still recognize it as a manifest file.
TL;DR
The manifest file can have a .js, .json, or .webmanifest extension.
In theory, this worked because I could see the manifest in the inspector, however the PWA still bombed. The reason, I discovered, is that the manifest has to be linked in the <head> of your HTML file.
Problem 4: The manifest file has to be linked in the document head.
This looked like a dealbreaker for me because I didn’t have access to the document head via the CMS, but I found a very simple work around. I linked the manifest within the body, then gave the link tag an ID of “manifest”. That allowed me to easily use an inline JavaScript to move it into the head:
const mani = document.getElementById("manifest");
document.head.appendChild(mani);
I had to set the two service worker scripts I was referencing below (the service worker JavaScript and the JavaScript to register the service worker and add the PWA functions) to “defer” to allow time for the manifest to be moved and the head re-parsed before the service worker scripts were run, but once that was done it actually worked, and so did my PWA.
TL;DR
The manifest file has to be in the HTML head, but it can be moved there after page load with JS and still work.
Now it’s time to actually make the PWA do something! I’m sure that’s going to be another long post.